Connecting to Boards
Edit this on GitLab
Connecting to Products using the NAI Software Support Kit (SSK).
To download the NAI Software Support Kit click here.
The naibrd SSK is an integrated software package that provides support the Generation 5 NAI boards and some Generation 3 NAI boards.
NAI Board Access
Depending on the system, access to the NAI boards can be configured as follows:
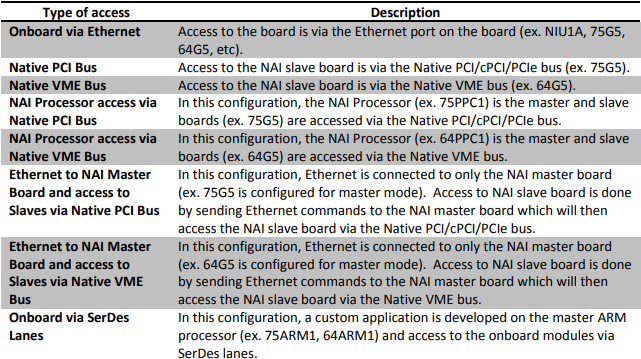
Onboard Via Ethernet
This applies to configurations where access to the NAI slave board is via the Ethernet port on the board (ex. NIU1A, 75G5, 64G5, etc).
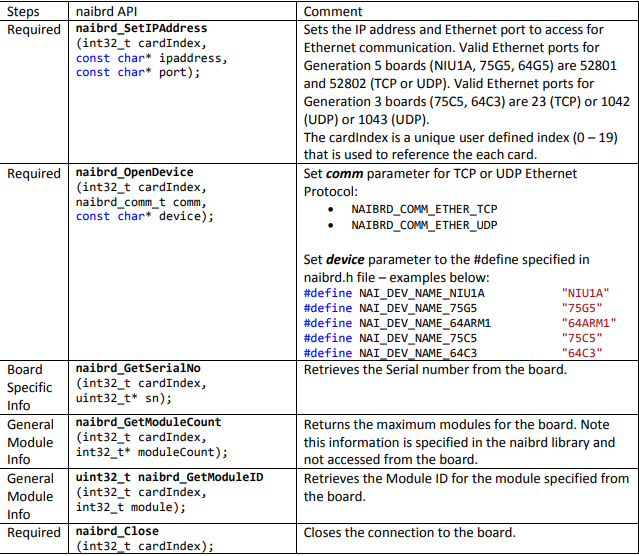
Example:
define NIU1A_IDX 1 /* Card index (0 – 19) */ #define IP_ADDR "10.0.8.123" #define IP_PORT "52801" /* 52801 or 52802 */ void sample (void) { uint32_t unSerialNum = 0; /* Set the IP address and Port of the card */ naibrd_SetIPAddress (NIU1A_IDX, IP_ADDR, IP_PORT); /* Open the card specifying Ethernet protocol and device name */ naibrd_OpenDevice (NIU1A_IDX, NAIBRD_COMM_ETHER_TCP, NAI_DEV_NAME_NIU1A); /* Get and display the card serial number */ naibrd_GetSerialNo (NIU1A_IDX, &unSerialNum); printf("Card:%d - Serial Number:%d\n", NIU1A_IDX, unSerialNum); /* Close the card */ naibrd_Close(NIU1A_IDX); }
Native PCI/cPCI/PCIe Bus
This applies to configurations where access to the NAI slave board (ex. 75G5) is via the Native PCI/cPCI/PCIe bus.
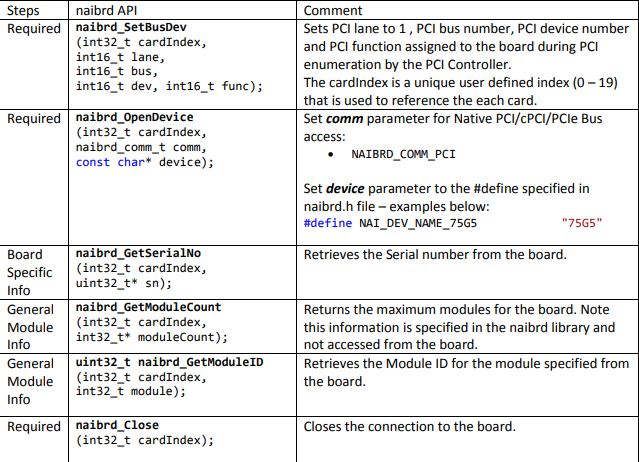
Example:
#define NAI75G5_IDX 1 /* Card index (0 – 19) */ #define PCI_LANE 1 #define PCI_BUS 2 #define PCI_DEV 14 #define PCI_FNC 0 void sample (void) { uint32_t unSerialNum = 0; /* Set the Lane, Bus, Device and Function of the card */ naibrd_SetBusDev (NAI75G5_IDX, PCI_LANE, PCI_BUS, PCI_DEV, PCI_FNC); /* Open the card specifying PCI bus and device name */ naibrd_OpenDevice (NAI75G5_IDX, NAIBRD_COMM_PCI, NAI_DEV_NAME_75G5); /* Get and display the card serial number */ naibrd_GetSerialNo (NAI75G5_IDX, &unSerialNum); printf("Card:%d - Serial Number:%d\n", NAI75G5_IDX, unSerialNum); /* Close the card */ naibrd_Close(NAI75G5_IDX); }
Native VME Bus
This applies to configurations where access to the NAI slave board (ex. 64G5) is via the Native VME bus.
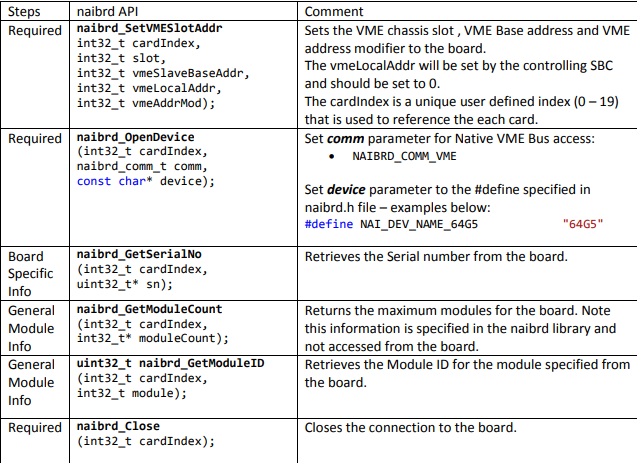
Example:
#define NAI64G5_IDX 1 /* Card index (0 – 19) */ #define VME_SLOT 3 /* Slot in VME Frame the card occupies */ #define VME_BASE_ADDRESS 0x01000000 /* VME Base Address of card */ #define VME_ADDR_MOD 0x0D /* VME Address Modifier */ void sample (void) { uint32_t unSerialNum = 0; /* Set the Slot, VME Address and Modifier of the card */ naibrd_SetVMESlotAddr (NAI64G5_IDX, VME_SLOT, VME_BASE_ADDRESS, 0, VME_ADDR_MOD); /* Open the card specifying VME bus and device name */ naibrd_OpenDevice (NAI64G5_IDX, NAIBRD_COMM_VME, NAI_DEV_NAME_64G5); /* Get and display the card serial number */ naibrd_GetSerialNo (NAI64G5_IDX, &unSerialNum); printf("Card:%d - Serial Number:%d\n", NAI64G5_IDX, unSerialNum); /* Close the card */ naibrd_Close(NAI64G5_IDX); }
NAI Processor access via Native PCI/cPCI/PCIe Bus
This applies to configurations where the system contains a board with a NAI Processor (ex. 75PPC1) configured as the master controller, and slave boards (ex. 75G5) are accessed via the Native PCI/cPCI/PCIe bus.
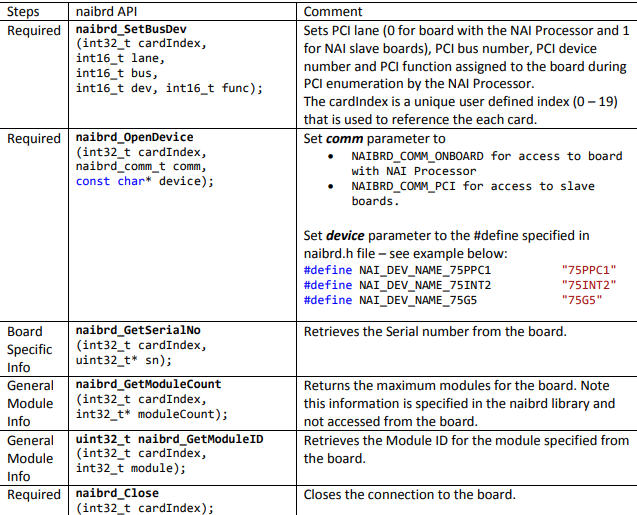
Example:
#define NAI75PPC1_IDX 0 /* Card index (0 – 19) */ #define MASTER_PCI_LANE 0 /* Lane 0 - on board, Lane 1 - off board */ #define MASTER_PCI_BUS 1 #define MASTER_PCI_DEV 0 #define MASTER_PCI_FNC 0 #define NAI75G5_IDX 1 #define SLAVE_PCI_LANE 1 #define SLAVE_PCI_BUS 2 #define SLAVE_PCI_DEV 14 #define SLAVE_PCI_FNC 0 void sample (void) { uint32_t unSerialNum = 0; /* Master Card */ /* Set the Lane, Bus, Device and Function of the card */ naibrd_SetBusDev (NAI75PPC1_IDX, MASTER_PCI_LANE, MASTER_PCI_BUS, MASTER_PCI_DEV, MASTER_PCI_FNC); /* Open the card specifying bus and device name */ naibrd_OpenDevice (NAI75PPC1_IDX, NAIBRD_COMM_ONBOARD, NAI_DEV_NAME_75PPC1); /* Get and display the card serial number */ naibrd_GetSerialNo (NAI75PPC1_IDX, &unSerialNum); printf("Card:%d - Serial Number:%d\n", NAI75PPC1_IDX, unSerialNum); /* Slave Card */ /* Set the Lane, Bus, Device and Function of the card */ naibrd_SetBusDev (NAI75G5_IDX, SLAVE_PCI_LANE, SLAVE_PCI_BUS, SLAVE_PCI_DEV, SLAVE_PCI_FNC); /* Open the card specifying bus and device name */ naibrd_OpenDevice (NAI75G5_IDX, NAIBRD_COMM_PCI, NAI_DEV_NAME_75G5); /* Get and display the card serial number */ naibrd_GetSerialNo (NAI75G5_IDX, &unSerialNum); printf("Card:%d - Serial Number:%d\n", NAI75G5_IDX, unSerialNum); /* Close the cards */ naibrd_Close(NAI75PPC1_IDX); naibrd_Close(NAI75G5_IDX); }
NAI Processor access via Native VME Bus
This applies to configurations where the system contains a board with a NAI Processor (ex. 64PPC1) configured as the master controller, and slave boards (ex. 64G5) are accessed via the Native VME bus.
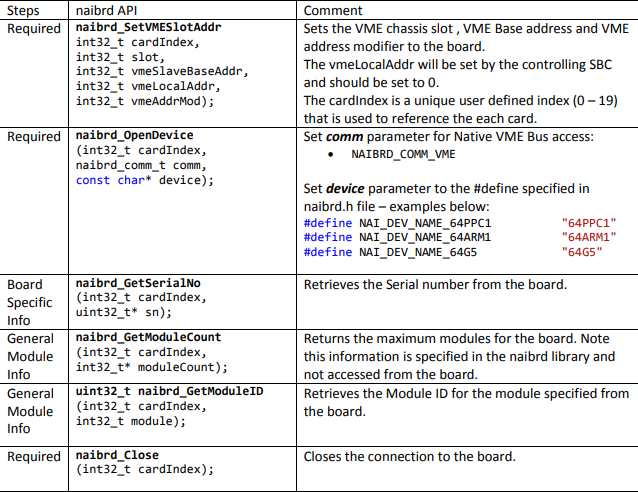
Example:
#define NAI64PPC1_IDX 0 /* Card index (0 – 19) */ #define NAI64ARM1_IDX 1 #define VME_SLOT 3 /* Slot in VME Frame the card occupies */ #define VME_BASE_ADDRESS 0x01000000 /* VME Base Address of card */ #define VME_ADDR_MOD 0x0D /* VME Address Modifier */ void sample (void) { uint32_t unSerialNum = 0; /* Open the NAI SBC for on board access */ naibrd_64PPC1_Open(NAI64PPC1_IDX, NAIBRD_COMM_ONBOARD); /* Get and display the card serial number */ naibrd_GetSerialNo (NAI64PPC1_IDX, &unSerialNum); printf("Card:%d - Serial Number:%d\n", NAI64PPC1_IDX, unSerialNum); /* Set the Slot, VME Address and Modifier of the slave card */ naibrd_SetVMESlotAddr (NAI64G5_IDX, VME_SLOT, VME_BASE_ADDRESS, 0, VME_ADDR_MOD); /* Open the slave card specifying VME bus and device name */ naibrd_OpenDevice (NAI64G5_IDX, NAIBRD_COMM_VME, NAI_DEV_NAME_64G5); /* Get and display the card serial number */ naibrd_GetSerialNo (NAI64G5_IDX, &unSerialNum); printf("Card:%d - Serial Number:%d\n", NAI64G5_IDX, unSerialNum); /* Close the cards */ naibrd_Close(NAI64PPC1_IDX); naibrd_Close(NAI64G5_IDX); }
Ethernet to NAI Master Board and access to slaves via Native PCI/cPCI/PCIe Bus
This applies to configurations where Ethernet is connected to only the NAI master board (ex. 75G5 in configured for master mode). Access to NAI slave board is done by sending Ethernet commands to the NAI master board which will then access the NAI slave board via the Native PCI/cPCI/PCIe bus.
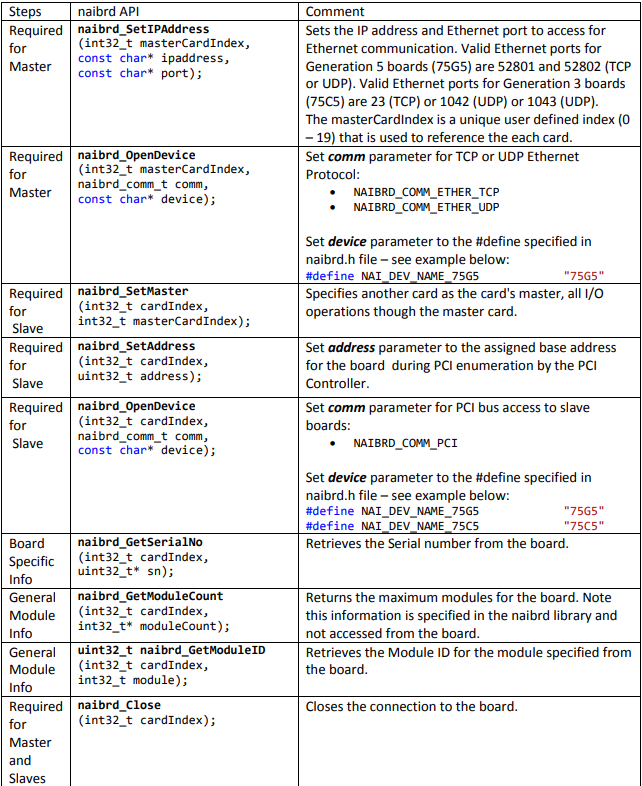
Example:
#define NAI75G5_MASTER_IDX 1 /* Card index (0 – 19) */ #define NAI75G5_SLAVE_IDX 2 #define NAI75G5_SLAVE_ADDR 0xA4104000 /* PCI Base Address of Slave Card */ #define IP_ADDR "10.0.8.123" #define IP_PORT "52801" /* 52801 or 52802 */ void sample (void) { uint32_t unSerialNum = 0; /* Set the IP address and Port of the card */ naibrd_SetIPAddress (NAI75G5_MASTER_IDX, IP_ADDR, IP_PORT); /* Open the card specifying Ethernet protocol and device name */ naibrd_OpenDevice (NAI75G5_MASTER_IDX, NAIBRD_COMM_ETHER_TCP, NAI_DEV_NAME_75G5); /* Set the Card as a "Master" to enable pass through to Slave on Bus */ naibrd_SetMaster(NAI75G5_SLAVE_IDX, NAI75G5_MASTER_IDX); /* Set the Base address of the Slave Card */ naibrd_SetAddress(NAI75G5_SLAVE_IDX, NAI75G5_SLAVE_ADDR); /* Open the card specifying PCI bus and device name */ naibrd_OpenDevice (NAI75G5_SLAVE_IDX, NAIBRD_COMM_PCI, NAI_DEV_NAME_75G5); /* Get and display the card serial number */ naibrd_GetSerialNo (NAI75G5_SLAVE_IDX, &unSerialNum); printf("Card:%d - Serial Number:%d\n", NAI75G5_SLAVE_IDX, unSerialNum); /* Close the cards */ naibrd_Close(NAI75G5_MASTER_IDX); naibrd_Close(NAI75G5_SLAVE_IDX); }
Ethernet to NAI Master Board and access to slaves via Native VME Bus
This applies to configurations where Ethernet is connected to only the NAI master board (ex. 64G5 in configured for master mode). Access to NAI slave board is done by sending Ethernet commands to the NAI master board which will then access the NAI slave board via the Native VME bus.
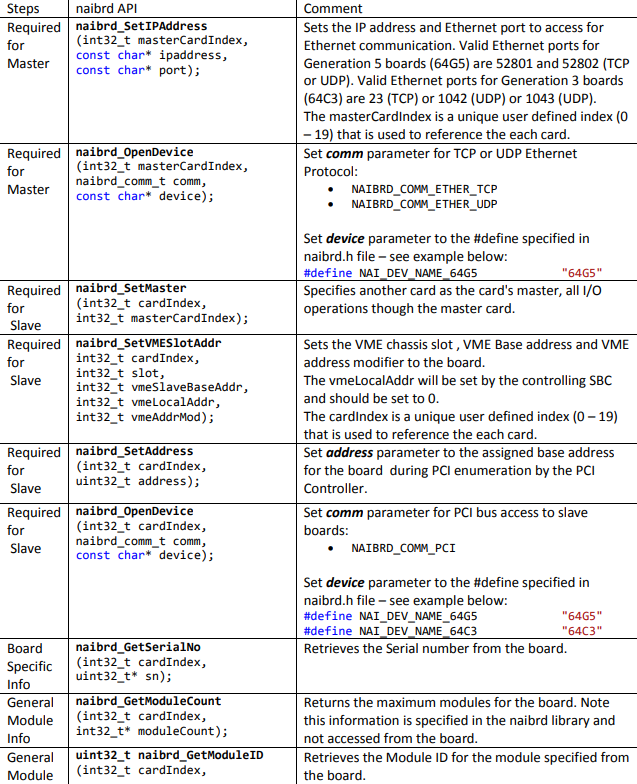
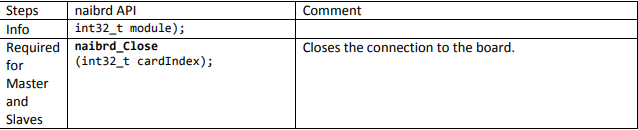
Example:
#define NAI64G5_MASTER_IDX 1 /* Card index (0 – 19) */ #define NAI64G5_SLAVE_IDX 2 #define VME_SLOT 3 /* Slot in VME Frame the card occupies */ #define VME_BASE_ADDRESS 0x01000000 /* VME Base Address of card */ #define VME_ADDR_MOD 0x0D /* VME Address Modifier */ #define IP_ADDR "10.0.8.123" #define IP_PORT "52801" /* 52801 or 52802 */ void sample (void) { uint32_t unSerialNum = 0; /* Set the IP address and Port of the card */ naibrd_SetIPAddress (NAI64G5_MASTER_IDX, IP_ADDR, IP_PORT); /* Open the card specifying Ethernet protocol and device name */ naibrd_OpenDevice (NAI64G5_MASTER_IDX, NAIBRD_COMM_ETHER_TCP, NAI_DEV_NAME_64G5); /* Set the Card as a "Master" to enable pass through to Slave on Bus */ naibrd_SetMaster(NAI64G5_SLAVE_IDX, NAI64G5_MASTER_IDX); /* Set the Slot, VME Address and Modifier of the slave card */ naibrd_SetVMESlotAddr (NAI64G5_SLAVE_IDX, VME_SLOT, VME_BASE_ADDRESS, 0, VME_ADDR_MOD); /* Open the card specifying VME bus and device name */ naibrd_OpenDevice (NAI64G5_SLAVE_IDX, NAIBRD_COMM_VME, NAI_DEV_NAME_64G5); /* Get and display the card serial number */ naibrd_GetSerialNo (NAI64G5_SLAVE_IDX, &unSerialNum); printf("Card:%d - Serial Number:%d\n", NAI64G5_SLAVE_IDX, unSerialNum); /* Close the cards */ naibrd_Close(NAI64G5_MASTER_IDX); naibrd_Close(NAI64G5_SLAVE_IDX); }
Onboard via SerDes
This applies to configurations where a custom application is developed on the master ARM processor (ex. 75ARM1, 64ARM1, NIU1A) and access to the onboard modules via SerDes.
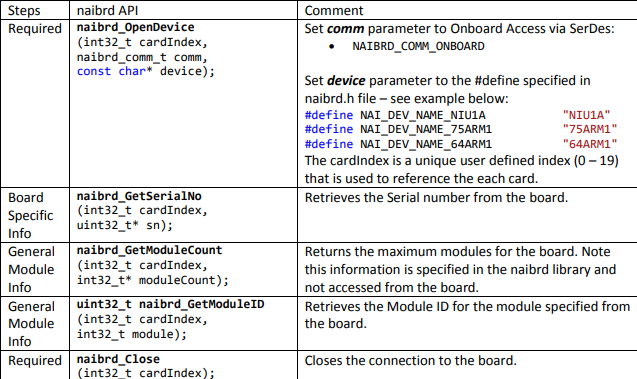
Example:
#define NAI64ARM1_IDX 0 /* Card index (0 – 19) */ #define MODULE_NUM 1 void sample (void) { uint32_t unModuleId = 0; /* Open the card specifying Onboard SERDES and device name */ naibrd_OpenDevice (NAI64ARM1_IDX, NAIBRD_COMM_ONBOARD, NAI_DEV_NAME_64ARM1); /* Get and display the ID of the first Module */ unModuleId = naibrd_GetModuleID (NAI64ARM1_IDX, MODULE_NUM); printf("Card:%d - Module %d ID:0x%X\n", NAI64ARM1_IDX, MODULE_NUM, unModuleId); /* Close the card */ naibrd_Close(NAI64ARM1_IDX); }